Chapter 2#
[1]:
from PIL import Image
import webgpupy as np
[2]:
def convert_to_image(pixels):
print(pixels.shape)
pixels = (pixels * 255.0).astype('uint8')
import numpy
image_array = numpy.array(pixels.tolist(), dtype= 'uint8')
return Image.fromarray(image_array, 'RGB')
[4]:
image_width = 640
image_height = 360
row = [(i/image_width) for i in reversed(range(0, image_width))]
column = [(i/image_height) for i in range(0, image_height)]
r = np.array(row).reshape([1, image_width])
g = np.array(column).reshape([image_height, 1])
b = np.full([image_height, image_width], 0.25)
r = np.repeat(r, image_height, axis=0)
g = np.repeat(g, image_width, axis=1)
pixels = np.dstack([r,g,b])
convert_to_image(pixels)
[360, 640, 3]
[4]:
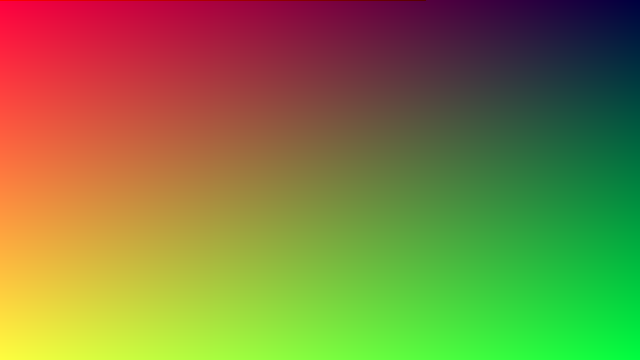